It's a simple File Explorer in Android.
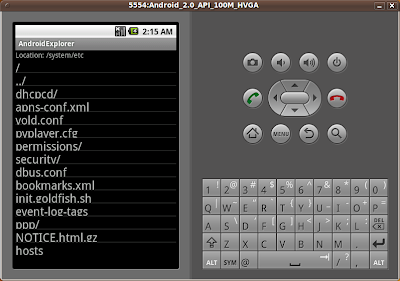
The files information can be read using java.io.File, using the code:
File f = new File(dirPath);
File[] files = f.listFiles();
The name of the files/folders will be added in a ArrayList. If it's not the root, two more elements (the root and the parent) will be added in the front of the ArrayList.
Then, the ArrayList will be adapted to a ArrayAdapter, and also set to be displayed on screen.
In case any item is clicked; if it's a file, the name will be display in a dialog; if it's a directory (isDirectory) and can be read (canRead), will open the selected directory; if it's a directory and CANNOT be read, a message will be prompt.


Create a layout file /res/layout/row.xml, which is the layout of each row the the list.
Modify /res/layout/main.xml to have a List:
remark: TextView with id empty will be display IF the list is empty.
Modify the code, AndroidExplorer.java
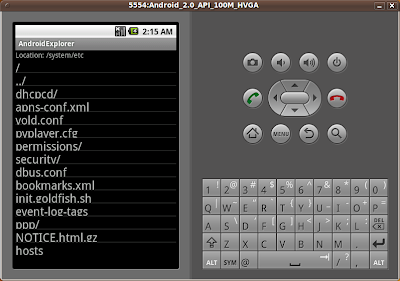
The files information can be read using java.io.File, using the code:
File f = new File(dirPath);
File[] files = f.listFiles();
The name of the files/folders will be added in a ArrayList. If it's not the root, two more elements (the root and the parent) will be added in the front of the ArrayList.
Then, the ArrayList will be adapted to a ArrayAdapter, and also set to be displayed on screen.
In case any item is clicked; if it's a file, the name will be display in a dialog; if it's a directory (isDirectory) and can be read (canRead), will open the selected directory; if it's a directory and CANNOT be read, a message will be prompt.


Create a layout file /res/layout/row.xml, which is the layout of each row the the list.
<?xml version="1.0" encoding="utf-8"?>
<TextView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/rowtext"
android:layout_width="fill_parent"
android:layout_height="25px"
android:textSize="23sp" />
Modify /res/layout/main.xml to have a List:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:id="@+id/path"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<ListView
android:id="@android:id/list"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<TextView
android:id="@android:id/empty"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="No Data"
/>
</LinearLayout>
remark: TextView with id empty will be display IF the list is empty.
Modify the code, AndroidExplorer.java
package com.AndroidExplorer;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
public class AndroidExplorer extends ListActivity {
private List<String> item = null;
private List<String> path = null;
private String root="/";
private TextView myPath;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
myPath = (TextView)findViewById(R.id.path);
getDir(root);
}
private void getDir(String dirPath)
{
myPath.setText("Location: " + dirPath);
item = new ArrayList<String>();
path = new ArrayList<String>();
File f = new File(dirPath);
File[] files = f.listFiles();
if(!dirPath.equals(root))
{
item.add(root);
path.add(root);
item.add("../");
path.add(f.getParent());
}
for(int i=0; i < files.length; i++)
{
File file = files[i];
path.add(file.getPath());
if(file.isDirectory())
item.add(file.getName() + "/");
else
item.add(file.getName());
}
ArrayAdapter<String> fileList =
new ArrayAdapter<String>(this, R.layout.row, item);
setListAdapter(fileList);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
File file = new File(path.get(position));
if (file.isDirectory())
{
if(file.canRead())
getDir(path.get(position));
else
{
new AlertDialog.Builder(this)
.setIcon(R.drawable.icon)
.setTitle("[" + file.getName() + "] folder can't be read!")
.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
}
}).show();
}
}
else
{
new AlertDialog.Builder(this)
.setIcon(R.drawable.icon)
.setTitle("[" + file.getName() + "]")
.setPositiveButton("OK",
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
}
}).show();
}
}
}
No comments :
Post a Comment