Android Expandable ListView Example
The Android Development Tutorials blog contains Basic as well as Advanced android tutorials.Go to Android Development Tutorials to get list of all Android Tutorials.
Level 1 contains Parent items and Level 2 contains Child items. The items are filled/populated from the ExpandableListAdapter associated with the Parent and Child view.
In below snapshot Fruits, Flowers, Animals, Birds are Parent Items and Apple,Mango,Banana,Orange,Lion,Tiger etc are Child Items.
ExpandableListView
is a view that shows items in a vertically scrolling two-level list. This differs from the ListView by allowing two levels: groups which can individually be expanded to show its children.Level 1 contains Parent items and Level 2 contains Child items. The items are filled/populated from the ExpandableListAdapter associated with the Parent and Child view.
In below snapshot Fruits, Flowers, Animals, Birds are Parent Items and Apple,Mango,Banana,Orange,Lion,Tiger etc are Child Items.
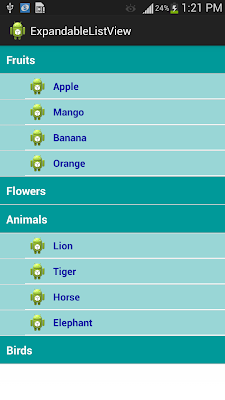
ExpandableListView Example
In this example we will learn how to use ExpandableListView and how to populate it's item through ExpandableListAdapterSteps:
1: Create xml for Parent Item
2: Create xml for Child Item
3: Create MainActivity and populate the data for Parent and Child Items
4: Create ExpandableListAdapter class and implement getGroupView and getChildView methods according to your need.
Also add clickListener in getChildView method to handle click events on child items.
Step1:
parent_view.xml
<CheckedTextView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/textViewGroupName"
android:layout_width="wrap_content"
android:layout_height="60dp"
android:layout_marginLeft="5dp"
android:gravity="center_vertical"
android:text="@string/hello_world"
android:textSize="18dp"
android:textColor="#FFFFFF"
android:padding="10dp"
android:textSelectHandleLeft="@string/hello_world"
android:background="#009999"
android:textStyle="bold" />
Step 2:
child_view.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="40dp"
android:background="#99D6D6"
android:clickable="true"
android:orientation="vertical"
android:paddingLeft="40dp"
tools:context=".MainActivity" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="39dp"
android:gravity="center_vertical" >
<ImageView
android:id="@+id/childImage"
android:layout_width="30dp"
android:layout_height="30dp"
android:layout_margin="5dp"
android:background="@drawable/ic_launcher"
android:contentDescription="@string/hello_world" />
<TextView
android:id="@+id/textViewChild"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:text="@string/hello_world"
android:textSize="16sp"
android:textColor="#1919A3"
android:textStyle="bold" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@android:color/white" />
</LinearLayout>
Step 3:
ExpandableListMainActivity.java
public class ExpandableListMainActivity extends ExpandableListActivity
{
// Create ArrayList to hold parent Items and Child Items
private ArrayList<String> parentItems = new ArrayList<String>();
private ArrayList<Object> childItems = new ArrayList<Object>();
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
// Create Expandable List and set it's properties
ExpandableListView expandableList = getExpandableListView();
expandableList.setDividerHeight(2);
expandableList.setGroupIndicator(null);
expandableList.setClickable(true);
// Set the Items of Parent
setGroupParents();
// Set The Child Data
setChildData();
// Create the Adapter
MyExpandableAdapter adapter = new MyExpandableAdapter(parentItems, childItems);
adapter.setInflater((LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE), this);
// Set the Adapter to expandableList
expandableList.setAdapter(adapter);
expandableList.setOnChildClickListener(this);
}
// method to add parent Items
public void setGroupParents()
{
parentItems.add("Fruits");
parentItems.add("Flowers");
parentItems.add("Animals");
parentItems.add("Birds");
}
// method to set child data of each parent
public void setChildData()
{
// Add Child Items for Fruits
ArrayList<String> child = new ArrayList<String>();
child.add("Apple");
child.add("Mango");
child.add("Banana");
child.add("Orange");
childItems.add(child);
// Add Child Items for Flowers
child = new ArrayList<String>();
child.add("Rose");
child.add("Lotus");
child.add("Jasmine");
child.add("Lily");
childItems.add(child);
// Add Child Items for Animals
child = new ArrayList<String>();
child.add("Lion");
child.add("Tiger");
child.add("Horse");
child.add("Elephant");
childItems.add(child);
// Add Child Items for Birds
child = new ArrayList<String>();
child.add("Parrot");
child.add("Sparrow");
child.add("Peacock");
child.add("Pigeon");
childItems.add(child);
}
}
Step 4:
MyExpandableAdapter.java
public class MyExpandableAdapter extends BaseExpandableListAdapter
{
private Activity activity;
private ArrayList<Object> childtems;
private LayoutInflater inflater;
private ArrayList<String> parentItems, child;
// constructor
public MyExpandableAdapter(ArrayList<String> parents, ArrayList<Object> childern)
{
this.parentItems = parents;
this.childtems = childern;
}
public void setInflater(LayoutInflater inflater, Activity activity)
{
this.inflater = inflater;
this.activity = activity;
}
// method getChildView is called automatically for each child view.
// Implement this method as per your requirement
@Override
public View getChildView(int groupPosition, final int childPosition, boolean isLastChild, View convertView, ViewGroup parent)
{
child = (ArrayList<String>) childtems.get(groupPosition);
TextView textView = null;
if (convertView == null) {
convertView = inflater.inflate(R.layout.child_view, null);
}
// get the textView reference and set the value
textView = (TextView) convertView.findViewById(R.id.textViewChild);
textView.setText(child.get(childPosition));
// set the ClickListener to handle the click event on child item
convertView.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
Toast.makeText(activity, child.get(childPosition),
Toast.LENGTH_SHORT).show();
}
});
return convertView;
}
// method getGroupView is called automatically for each parent item
// Implement this method as per your requirement
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent)
{
if (convertView == null) {
convertView = inflater.inflate(R.layout.parent_view, null);
}
((CheckedTextView) convertView).setText(parentItems.get(groupPosition));
((CheckedTextView) convertView).setChecked(isExpanded);
return convertView;
}
@Override
public Object getChild(int groupPosition, int childPosition)
{
return null;
}
@Override
public long getChildId(int groupPosition, int childPosition)
{
return 0;
}
@Override
public int getChildrenCount(int groupPosition)
{
return ((ArrayList<String>) childtems.get(groupPosition)).size();
}
@Override
public Object getGroup(int groupPosition)
{
return null;
}
@Override
public int getGroupCount()
{
return parentItems.size();
}
@Override
public void onGroupCollapsed(int groupPosition)
{
super.onGroupCollapsed(groupPosition);
}
@Override
public void onGroupExpanded(int groupPosition)
{
super.onGroupExpanded(groupPosition);
}
@Override
public long getGroupId(int groupPosition)
{
return 0;
}
@Override
public boolean hasStableIds()
{
return false;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition)
{
return false;
}
}
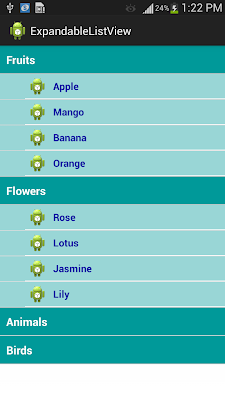
No comments :
Post a Comment