BROADCAST RECEIVER
TO REGISTER THE BROADCAST RECEIVER PROGRAMMATICALLY/DYNAMICALLY
I have statically registered MyBroadcastReceiver in the AndroinManifest.xml file. In this example I have used my own event ‘MyBroadcast’
Android broadcastreceiver is also a component where you can register for system or application events. You will be notified about the events after registering. Broadcast originates from the system as well as applications. Instance for broadcast originating from the system is ‘low battery notification’. Application level is, like when you download an mp3 file, Mp3 player gets notified about it, and gets added to player list. For this action to take place, mp3 player has to register for this event. Another simple example is, users will be notified when the battery goes below certain limit as shown below;
In this example you will know how to create custom broadcast receiver in android.
It means ACTION_BATTERY_LOW event has been registered and the Android system fires it when the battery level goes down. Incoming message notifications, wifi notifications all are broadcast receivers.
There are two ways to register Android broadcastreceiver.
We have to be careful enough while creating the dynamic broadcast receivers. If we forget to unregister the broadcast receiver, the system will report a leaked broadcast receiver error.
context is can be used to start services or activities and the intent is object with the action you used to register your receiver. This can contain additional information you can use in your implementation.
The broadcast receiver can statically be registered via AndroidManifest.xml as said earlier.
The elementis used to specify the event the receiver should react to. So whenever this event ‘MyBroadcast’ occurs MyBroadcastReceiver’s onReceive() method will be invoked.
As soon as the onReceive() method is finished, your BroadcastReceiver terminates.
SOME EVENTS MAY REQUIRE PERMISSIONS
For some events we may require permissions. For example to use
We need to add
Finally the AndroidManifest.xml file may look like;
In this example you will know how to create custom broadcast receiver in android.
It means ACTION_BATTERY_LOW event has been registered and the Android system fires it when the battery level goes down. Incoming message notifications, wifi notifications all are broadcast receivers.
There are two ways to register Android broadcastreceiver.
- One is static way in which the broadcast receiver is registered in an android application via AndroidManifest.xml file.
- Another way of registering the broadcast receiver is dynamic, which is done using Context.registerReceiver() method. Dynamically registered broadcast receivers can be unregistered using Context.unregisterReceiver() method.
We have to be careful enough while creating the dynamic broadcast receivers. If we forget to unregister the broadcast receiver, the system will report a leaked broadcast receiver error.
Create your Broadcast receiver and Implement onReceive() method
A broadcast receiver extends BroadcastReceiver abstract class. Which means that we have to implement the onReceive() method of this base class. Whenever the event occurs Android calls the onReceive() method on the registered broadcast receiver. For example, if you register for ACTION_POWER_CONNECTED event then whenever power got connected to the device, your broadcast receiver’s onReceive() method will be invoked.onReceive() Method
The onReceive() method takes two arguments. onReceive(Context context,Intent intent)context is can be used to start services or activities and the intent is object with the action you used to register your receiver. This can contain additional information you can use in your implementation.
Register your Broadcast Receiver
TO REGISTER THE BROADCAST RECEIVER STATICALLYThe broadcast receiver can statically be registered via AndroidManifest.xml as said earlier.
The element
As soon as the onReceive() method is finished, your BroadcastReceiver terminates.
SOME EVENTS MAY REQUIRE PERMISSIONS
For some events we may require permissions. For example to use
<action android:name="android.intent.action.PHONE_STATE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
Finally the AndroidManifest.xml file may look like;
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.broadcastreceiverdemo" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="16" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.broadcastreceiverdemo.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <receiver android:name="BroadCastReceiver_Name" > <intent-filter> <action android:name="Event_Name" > </action> </intent-filter> </receiver> </application> </manifest>
BroadcastReceiver mReceiver=new MyBroadcastReceiver(); registerReceiver( this.myReceiver, new IntentFilter("MyBroadcast"));Here 'this' is your current context.
Avoid long Running tasks
We should avoid long running tasks in Broadcast receivers. For any longer tasks we can start a service from within the receiver. Dynamically registered receivers are called on the UI thread. Dynamically registered receivers blocks any UI handling and thus the onReceive() method should be as fast as possible. The application may become sluggish of an “Application Not Responding” error is the worst.Sample Application
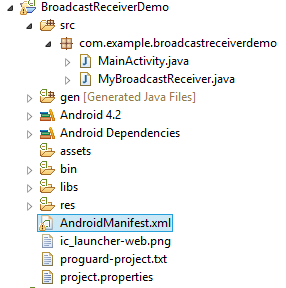
I have statically registered MyBroadcastReceiver in the AndroinManifest.xml file. In this example I have used my own event ‘MyBroadcast’
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.broadcastreceiverdemo" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="16" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.broadcastreceiverdemo.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <receiver android:name="MyBroadcastReceiver" > <intent-filter> <action android:name="MyBroadcast" > </action> </intent-filter> </receiver> </application> </manifest>I am sending the broadcast ‘MyBroadcast’ from my main activity as follows;
Intent intent = new Intent(); intent.setAction("MyBroadcast"); intent.putExtra("value", 1000); sendBroadcast(intent);Am sending the broadcast with an additional information ‘value=1000’. This additional information can be received by the broadcast receiver as follows;
Bundle extras = intent.getExtras(); if (extras != null) { if(extras.containsKey("value")){ String value=extras.get("value"); } }So my complete MainActivity.java is;
package com.example.broadcastreceiverdemo; import android.app.Activity; import android.content.Intent; import android.os.Bundle; /** * @author Prabu * */ public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Intent intent = new Intent(); intent.setAction("MyBroadcast"); intent.putExtra("value", 1000); sendBroadcast(intent); } }And my complete broadcast receiver implementation is;
/** * */ package com.example.broadcastreceiverdemo; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.os.Bundle; /** * @author Prabu * */ public class MyBroadcastReceiver extends BroadcastReceiver{ @Override public void onReceive(Context context, Intent intent) { Bundle extras = intent.getExtras(); if (extras != null) { if(extras.containsKey("value")){ System.out.println("Value is:"+extras.get("value")); } } } }
No comments :
Post a Comment