Preference Activity In Android Example
PreferenceActivity is the base class for an activity to show a hierarchy of preferences to the user.
It is generally used for saving the setting/preference of User. Android provides excellent framework to save and mange the User preferences.
We just need to use the class and specify some resources , and it automatically creates GUI for that itself.
In this example I have created a Page/Screen with user can save his password, and other choices/details.
1: Create xml resource for Preference: create an xml file inside xml folder (if xml folder is not there inside res folder, createxml folder inside res folder. In the example I have created user_settings.xml file inside xml folder.
2: Create a Class which extends PreferenceActivity class , and inflate the created xml using addPreferencesFromResource() method.
3: Call/Start the PreferenceActivity (created in 2nd steps) from where you want. In the example I have called from MainActivity using startActivityForResult() method.
Now lets go Step by Step:
user_settings.xml
This file defines user interface for setting page. We have created 3 different categories here.
1: EditTextPreference is used to define an editable text property. In this example used this field to enter the Password
2:CheckBoxPreference allows you to define a boolean value in preference screen.
3: ListPreference display choices from which user can select one.. In this examplewe have used ListPreference to choose the frequency when user should be reminded.
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen xmlns:android="http://schemas.android.com/apk/res/android" >
<PreferenceCategory android:title="Settings" >
<EditTextPreference
android:title="Password"
android:summary="Set Your Password"
android:key="prefUserPassword"/>
</PreferenceCategory>
<PreferenceCategory android:title="Security Settings" >
<CheckBoxPreference
android:defaultValue="false"
android:key="prefLockScreen"
android:summary="Lock The Screen With Password"
android:title="Screen Lock" >
</CheckBoxPreference>
<ListPreference
android:key="prefUpdateFrequency"
android:title="Reminder for Updation"
android:summary="Set Update Reminder Frequency"
android:entries="@array/updateFrequency"
android:entryValues="@array/updateFrequencyValues"
/>
</PreferenceCategory>
</PreferenceScreen>
Since we have used arrays here, We need to define it inside array.xml file.
Create an arrays.xml file inside values folder and write following
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="updateFrequency">
<item name="1">Daily</item>
<item name="7">Weekly</item>
<item name="3">Yearly</item>
<item name="0">Never(I will Myself) </item>
</string-array>
<string-array name="updateFrequencyValues">
<item name="1">1</item>
<item name="7">7</item>
<item name="30">30</item>
<item name="0">0</item>
</string-array>
</resources>
public class UserSettingActivity extends PreferenceActivity
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
// add the xml resource addPreferencesFromResource(R.xml.user_settings);
}
}
xml for MainActivity
main.xml contains two things
1: Button: We start UserSettingActivity when user clicks on this buttons.
2: TextView: To show the User data like password etc.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#CCCCFF"
tools:context=".MainActivity" >
<Button
android:id="@+id/buttonSettings"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="150dp"
android:textSize="25dp"
android:text="Settings" />
<TextView
android:id="@+id/textViewSettings"
android:textSize="21dp"
android:textColor="#0000FF"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_below="@+id/buttonSettings"
android:layout_marginTop="66dp"
android:text="No Settings"
android:textAppearance="?android:attr/textAppearanceMedium" />
</RelativeLayout>
MainActivty
public class MainActivity extends Activity
{
private static final int SETTINGS_RESULT = 1;
Button settingButton;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button btnSettings=(Button)findViewById(R.id.buttonSettings);
// start the UserSettingActivity when user clicks on Button
btnSettings.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// TODO Auto-generated method stub
Intent i = new Intent(getApplicationContext(), UserSettingActivity.class);
startActivityForResult(i, SETTINGS_RESULT);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
{
super.onActivityResult(requestCode, resultCode, data);
if(requestCode==SETTINGS_RESULT)
{
displayUserSettings();
}
}
private void displayUserSettings()
{
SharedPreferences sharedPrefs = PreferenceManager.getDefaultSharedPreferences(this);
String settings = "";
settings=settings+"Password: " + sharedPrefs.getString("prefUserPassword", "NOPASSWORD");
settings=settings+"\nRemind For Update:"+ sharedPrefs.getBoolean("prefLockScreen", false);
settings=settings+"\nUpdate Frequency: "
+ sharedPrefs.getString("prefUpdateFrequency", "NOUPDATE");
TextView textViewSetting = (TextView) findViewById(R.id.textViewSettings);
textViewSetting.setText(settings);
}
}
sharedPrefs.getString("prefUserPassword", "NOPASSWORD");
we need to provide the key , that was given in xml ( android:key="prefUserPassword" in user_settings.xml file )
We need to pass key as first argument and default value as second argumment.
It is generally used for saving the setting/preference of User. Android provides excellent framework to save and mange the User preferences.
We just need to use the class and specify some resources , and it automatically creates GUI for that itself.
PreferenceActivity Example In Android:
In this example I have created a Page/Screen with user can save his password, and other choices/details.
Steps:
1: Create xml resource for Preference: create an xml file inside xml folder (if xml folder is not there inside res folder, createxml folder inside res folder. In the example I have created user_settings.xml file inside xml folder.
2: Create a Class which extends PreferenceActivity class , and inflate the created xml using addPreferencesFromResource() method.
3: Call/Start the PreferenceActivity (created in 2nd steps) from where you want. In the example I have called from MainActivity using startActivityForResult() method.
Now lets go Step by Step:
Step1: Create XML resource inside xml folder:
user_settings.xml
This file defines user interface for setting page. We have created 3 different categories here.
1: EditTextPreference is used to define an editable text property. In this example used this field to enter the Password
2:CheckBoxPreference allows you to define a boolean value in preference screen.
3: ListPreference display choices from which user can select one.. In this examplewe have used ListPreference to choose the frequency when user should be reminded.
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen xmlns:android="http://schemas.android.com/apk/res/android" >
<PreferenceCategory android:title="Settings" >
<EditTextPreference
android:title="Password"
android:summary="Set Your Password"
android:key="prefUserPassword"/>
</PreferenceCategory>
<PreferenceCategory android:title="Security Settings" >
<CheckBoxPreference
android:defaultValue="false"
android:key="prefLockScreen"
android:summary="Lock The Screen With Password"
android:title="Screen Lock" >
</CheckBoxPreference>
<ListPreference
android:key="prefUpdateFrequency"
android:title="Reminder for Updation"
android:summary="Set Update Reminder Frequency"
android:entries="@array/updateFrequency"
android:entryValues="@array/updateFrequencyValues"
/>
</PreferenceCategory>
</PreferenceScreen>
Since we have used arrays here, We need to define it inside array.xml file.
Create an arrays.xml file inside values folder and write following
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="updateFrequency">
<item name="1">Daily</item>
<item name="7">Weekly</item>
<item name="3">Yearly</item>
<item name="0">Never(I will Myself) </item>
</string-array>
<string-array name="updateFrequencyValues">
<item name="1">1</item>
<item name="7">7</item>
<item name="30">30</item>
<item name="0">0</item>
</string-array>
</resources>
2: Create a Class which extends PreferenceActivity class
public class UserSettingActivity extends PreferenceActivity
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
// add the xml resource addPreferencesFromResource(R.xml.user_settings);
}
}
3: Call/Start the PreferenceActivity.
We have called UserSettingActivity from MainActivityxml for MainActivity
main.xml contains two things
1: Button: We start UserSettingActivity when user clicks on this buttons.
2: TextView: To show the User data like password etc.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#CCCCFF"
tools:context=".MainActivity" >
<Button
android:id="@+id/buttonSettings"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="150dp"
android:textSize="25dp"
android:text="Settings" />
<TextView
android:id="@+id/textViewSettings"
android:textSize="21dp"
android:textColor="#0000FF"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_below="@+id/buttonSettings"
android:layout_marginTop="66dp"
android:text="No Settings"
android:textAppearance="?android:attr/textAppearanceMedium" />
</RelativeLayout>
MainActivty
public class MainActivity extends Activity
{
private static final int SETTINGS_RESULT = 1;
Button settingButton;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button btnSettings=(Button)findViewById(R.id.buttonSettings);
// start the UserSettingActivity when user clicks on Button
btnSettings.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// TODO Auto-generated method stub
Intent i = new Intent(getApplicationContext(), UserSettingActivity.class);
startActivityForResult(i, SETTINGS_RESULT);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
{
super.onActivityResult(requestCode, resultCode, data);
if(requestCode==SETTINGS_RESULT)
{
displayUserSettings();
}
}
private void displayUserSettings()
{
SharedPreferences sharedPrefs = PreferenceManager.getDefaultSharedPreferences(this);
String settings = "";
settings=settings+"Password: " + sharedPrefs.getString("prefUserPassword", "NOPASSWORD");
settings=settings+"\nRemind For Update:"+ sharedPrefs.getBoolean("prefLockScreen", false);
settings=settings+"\nUpdate Frequency: "
+ sharedPrefs.getString("prefUpdateFrequency", "NOUPDATE");
TextView textViewSetting = (TextView) findViewById(R.id.textViewSettings);
textViewSetting.setText(settings);
}
}
How to fetch the User Saved data:
sharedPrefs.getString("prefUserPassword", "NOPASSWORD");
we need to provide the key , that was given in xml ( android:key="prefUserPassword" in user_settings.xml file )
We need to pass key as first argument and default value as second argumment.
OUTPUT :-
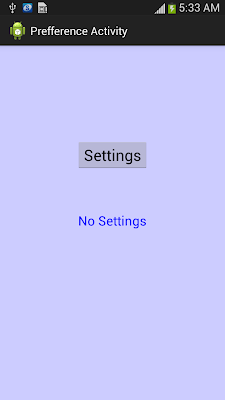
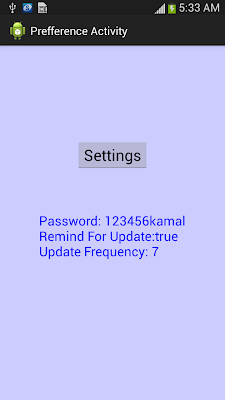
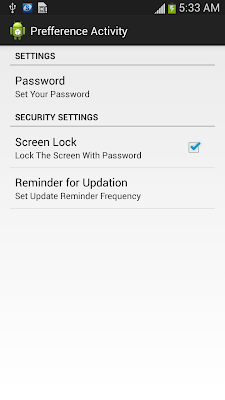
SHARE ON ARTICLE....
No comments :
Post a Comment