How to Create Option Menu In Android
Android Tutorial
Option Menu:
Option Menu is a drop down menu containing options, and appears when a users clicks on Menu button.For Ex:
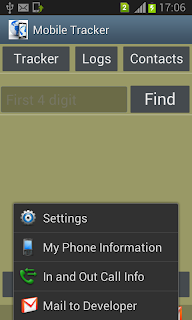
We can create an Option Menu with following :
Create a new folder named "menu" in res folder (if menu folder is not there in res folder)
inside this Menu folder create .xml file
here I have created option.xml xml for the option menu in above Image
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item android:id="@+id/ChangeColor"
android:icon="@drawable/setting"
android:title="Settings"
/>
<item android:id="@+id/phoneInformation"
android:icon="@drawable/phone"
android:title="My Phone Information" />
<item android:id="@+id/callInfo"
android:icon="@drawable/callinfo"
android:title="In and Out Call Info" />
<item android:id="@+id/email"
android:icon="@drawable/mail"
android:title="Mail to Developer" />
</menu>
Override onCreateOptionMenu method and inflate the .xml (here options.xml) inside method
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.options, menu);
return true;
}
When the user selects an item from the options menu (including action items in the action bar), the system calls your activity's
To Handle click events override onOptionsItemSelected method
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle item selection
switch (item.getItemId()) {
case R.id.ChangeColor:
// write code to execute when clicked on this option
return true;
case R.id.phoneInformation:
// write code to execute when clicked on this option
return true;
case R.id.callInfo:
// write code to execute when clicked on this option
return true;
case R.id.email:
// write code to execute when clicked on this option
return true;
default:
return super.onOptionsItemSelected(item);
}
}
public classMainActivity extends Activity
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
// Create Option Menu
@Override
public boolean onCreateOptionsMenu(Menu menu)
{
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.options, menu);
return true;
}
// Handle click events
@Override
public boolean onOptionsItemSelected(MenuItem item)
{
// Handle item selection
switch (item.getItemId()) {
case R.id.ChangeColor:
// write code to execute when clicked on this option
return true;
case R.id.phoneInformation:
// write code to execute when clicked on this option
return true;
case R.id.callInfo:
// write code to execute when clicked on this option
return true;
case R.id.email:
// write code to execute when clicked on this option
return true;
default:
return super.onOptionsItemSelected(item);
}
}
}
Activity Life Cycle
Starting Activity For Result
Sending Data from One Activity to Other in Android
Returning Result from Activity
Using CheckBoxes in Android
Using AutoCompleteTextView in Android
Adding Radio Buttons In Dialog
Adding Check Boxes In Dialog
Creating Customized Dialogs in Android
Adding EditText in Dialog
Creating Dialog To Collect User Input
How To Receive SMS
Accessing Inbox In Android
Creating Context Menu In Android
How to Forward an Incoming Call In Android
CALL States In Android
How To Vibrate The Android Phone
Sending Email In Android
Opening a webpage In Browser
How to Access PhoneBook In Android
Prompt User Input with an AlertDialog
Reading and Writing files to SD Card
Creating Table In Android
Inserting, Deleting and Updating Records In Table in Android
How to Create DataBase in Android
Accessing Inbox In Android
- Create a menu xml
- Register the menu in Activity
- Write code to Handle the Clicks on menu items
1: Create xml for menu
Create a new folder named "menu" in res folder (if menu folder is not there in res folder)
inside this Menu folder create .xml file
here I have created option.xml xml for the option menu in above Image
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item android:id="@+id/ChangeColor"
android:icon="@drawable/setting"
android:title="Settings"
/>
<item android:id="@+id/phoneInformation"
android:icon="@drawable/phone"
android:title="My Phone Information" />
<item android:id="@+id/callInfo"
android:icon="@drawable/callinfo"
android:title="In and Out Call Info" />
<item android:id="@+id/email"
android:icon="@drawable/mail"
android:title="Mail to Developer" />
</menu>
android:id
- A resource ID that's unique to the item, which allows the application can recognize the item when the user selects it.
android:icon
- An image to use as the item's icon.
android:title
- A tittle to show.
2: Register In Activity
Override onCreateOptionMenu method and inflate the .xml (here options.xml) inside method
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.options, menu);
return true;
}
3: Handle Click Events
When the user selects an item from the options menu (including action items in the action bar), the system calls your activity's
onOptionsItemSelected()
method. This method passes the MenuItem
selected. You can identify the item by callinggetItemId()
, which returns the unique ID for the menu item (defined by the android:id
attribute in the menu resource To Handle click events override onOptionsItemSelected method
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle item selection
switch (item.getItemId()) {
case R.id.ChangeColor:
// write code to execute when clicked on this option
return true;
case R.id.phoneInformation:
// write code to execute when clicked on this option
return true;
case R.id.callInfo:
// write code to execute when clicked on this option
return true;
case R.id.email:
// write code to execute when clicked on this option
return true;
default:
return super.onOptionsItemSelected(item);
}
}
Option Menu Full Source Code
public classMainActivity extends Activity
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
// Create Option Menu
@Override
public boolean onCreateOptionsMenu(Menu menu)
{
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.options, menu);
return true;
}
// Handle click events
@Override
public boolean onOptionsItemSelected(MenuItem item)
{
// Handle item selection
switch (item.getItemId()) {
case R.id.ChangeColor:
// write code to execute when clicked on this option
return true;
case R.id.phoneInformation:
// write code to execute when clicked on this option
return true;
case R.id.callInfo:
// write code to execute when clicked on this option
return true;
case R.id.email:
// write code to execute when clicked on this option
return true;
default:
return super.onOptionsItemSelected(item);
}
}
}
More Android Topics
Activity
Activity In AndroidActivity Life Cycle
Starting Activity For Result
Sending Data from One Activity to Other in Android
Returning Result from Activity
Working With Layouts
Understanding Layouts in AndroidWorking With Views
Using Buttons and EditText in AndroidUsing CheckBoxes in Android
Using AutoCompleteTextView in Android
Dialogs In Android
Working With Alert DialogAdding Radio Buttons In Dialog
Adding Check Boxes In Dialog
Creating Customized Dialogs in Android
Adding EditText in Dialog
Creating Dialog To Collect User Input
DatePicker and TimePickerDialog
Using TimePickerDialog and DatePickerDialog In androidWorking With SMS
How to Send SMS in AndroidHow To Receive SMS
Accessing Inbox In Android
ListView:
Populating ListView With DataBaseMenus In Android
Creating Option MenuCreating Context Menu In Android
TelephonyManager
Using Telephony Manager In AndroidWorking With Incoming Calls
How To Handle Incoming Calls in AndroidHow to Forward an Incoming Call In Android
CALL States In Android
Miscellaneous
Notifications In AndroidHow To Vibrate The Android Phone
Sending Email In Android
Opening a webpage In Browser
How to Access PhoneBook In Android
Prompt User Input with an AlertDialog
Storage: Storing Data In Android
Shared Prefferences In Android
SharedPreferences In AndroidFiles: File Handling In Android
Reading and Writing files to Internal StoarageReading and Writing files to SD Card
DataBase : Working With Database
Working With Database in AndroidCreating Table In Android
Inserting, Deleting and Updating Records In Table in Android
How to Create DataBase in Android
Accessing Inbox In Android
No comments :
Post a Comment