In this example uploading an image from sdcard to web server.
Steps :
1. place an image on sdcard.
2. place sdcard image path and image name in UploadToServer.java. ( see below )
3. create a php script (UploadToServer.php) at server. ( see below )
4. place php script path in UploadToServer.java. ( see below )
5. create folder name uploads on server where you have placed php script.
6. give permission 777 (read/write/execute) to uploads folder.
2. place sdcard image path and image name in UploadToServer.java. ( see below )
3. create a php script (UploadToServer.php) at server. ( see below )
4. place php script path in UploadToServer.java. ( see below )
5. create folder name uploads on server where you have placed php script.
6. give permission 777 (read/write/execute) to uploads folder.
Project Structure :
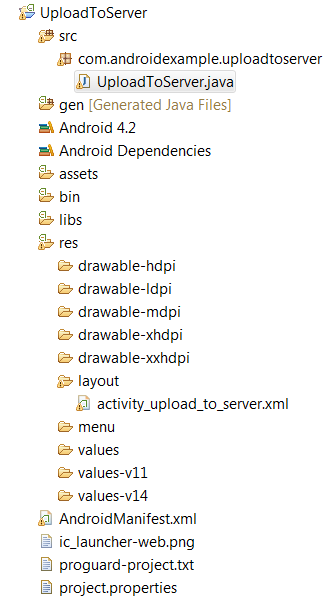
Upload Image Sdcard View :
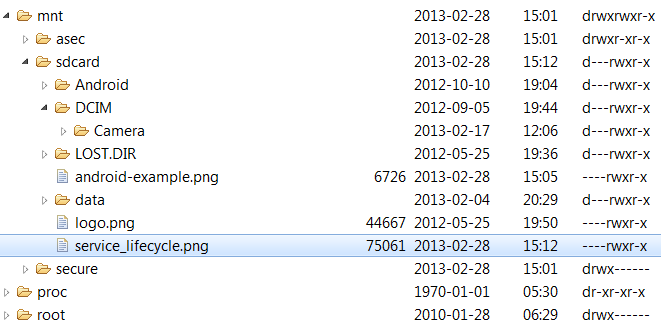
File : AndroidManifest.xml
<? xml version = "1.0" encoding = "utf-8" ?> < manifest xmlns:android = "http://schemas.android.com/apk/res/android" package = "com.androidexample.uploadtoserver" android:versionCode = "1" android:versionName = "1.0" > < uses-sdk android:minSdkVersion = "8" /> < uses-permission android:name = "android.permission.INTERNET" /> < application android:allowBackup = "true" android:icon = "@drawable/ic_launcher" android:label = "@string/app_name" android:theme = "@style/AppTheme" > < activity android:name = "com.androidexample.uploadtoserver.UploadToServer" android:label = "@string/app_name" > < intent-filter > < action android:name = "android.intent.action.MAIN" /> < category android:name = "android.intent.category.LAUNCHER" /> </ intent-filter > </ activity > </ application > </ manifest > |
File : res/layout/activity_upload_to_server.xml
<? xml version = "1.0" encoding = "utf-8" ?> < LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android" android:orientation = "vertical" android:layout_width = "fill_parent" android:layout_height = "fill_parent" > < Button android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:text = "Click To Upload File" android:id = "@+id/uploadButton" /> < TextView android:layout_width = "fill_parent" android:layout_height = "wrap_content" android:text = "" android:id = "@+id/messageText" android:textColor = "#000000" android:textStyle = "bold" /> </ LinearLayout > |
File : src/UploadToServer.java
import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; import android.app.Activity; import android.app.ProgressDialog; import android.os.Bundle; import android.util.Log; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.TextView; import android.widget.Toast; public class UploadToServer extends Activity { TextView messageText; Button uploadButton; int serverResponseCode = 0 ; ProgressDialog dialog = null ; String upLoadServerUri = null ; /********** File Path *************/ final String uploadFilePath = "/mnt/sdcard/" ; final String uploadFileName = "service_lifecycle.png" ; @Override public void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.activity_upload_to_server); uploadButton = (Button)findViewById(R.id.uploadButton); messageText = (TextView)findViewById(R.id.messageText); messageText.setText( "Uploading file path :- '/mnt/sdcard/" +uploadFileName+ "'" ); /************* Php script path ****************/ upLoadServerUri = "http://www.androidexample.com/media/UploadToServer.php" ; uploadButton.setOnClickListener( new OnClickListener() { @Override public void onClick(View v) { dialog = ProgressDialog.show(UploadToServer. this , "" , "Uploading file..." , true ); new Thread( new Runnable() { public void run() { runOnUiThread( new Runnable() { public void run() { messageText.setText( "uploading started....." ); } }); uploadFile(uploadFilePath + "" + uploadFileName); } }).start(); } }); } public int uploadFile(String sourceFileUri) { String fileName = sourceFileUri; HttpURLConnection conn = null ; DataOutputStream dos = null ; String lineEnd = "\r\n" ; String twoHyphens = "--" ; String boundary = "*****" ; int bytesRead, bytesAvailable, bufferSize; byte [] buffer; int maxBufferSize = 1 * 1024 * 1024 ; File sourceFile = new File(sourceFileUri); if (!sourceFile.isFile()) { dialog.dismiss(); Log.e( "uploadFile" , "Source File not exist :" +uploadFilePath + "" + uploadFileName); runOnUiThread( new Runnable() { public void run() { messageText.setText( "Source File not exist :" +uploadFilePath + "" + uploadFileName); } }); return 0 ; } else { try { // open a URL connection to the Servlet FileInputStream fileInputStream = new FileInputStream(sourceFile); URL url = new URL(upLoadServerUri); // Open a HTTP connection to the URL conn = (HttpURLConnection) url.openConnection(); conn.setDoInput( true ); // Allow Inputs conn.setDoOutput( true ); // Allow Outputs conn.setUseCaches( false ); // Don't use a Cached Copy conn.setRequestMethod( "POST" ); conn.setRequestProperty( "Connection" , "Keep-Alive" ); conn.setRequestProperty( "ENCTYPE" , "multipart/form-data" ); conn.setRequestProperty( "Content-Type" , "multipart/form-data;boundary=" + boundary); conn.setRequestProperty( "uploaded_file" , fileName); dos = new DataOutputStream(conn.getOutputStream()); dos.writeBytes(twoHyphens + boundary + lineEnd); dos.writeBytes( "Content-Disposition: form-data; name=" uploaded_file ";filename=" " + fileName + "" " + lineEnd); dos.writeBytes(lineEnd); // create a buffer of maximum size bytesAvailable = fileInputStream.available(); bufferSize = Math.min(bytesAvailable, maxBufferSize); buffer = new byte [bufferSize]; // read file and write it into form... bytesRead = fileInputStream.read(buffer, 0 , bufferSize); while (bytesRead > 0 ) { dos.write(buffer, 0 , bufferSize); bytesAvailable = fileInputStream.available(); bufferSize = Math.min(bytesAvailable, maxBufferSize); bytesRead = fileInputStream.read(buffer, 0 , bufferSize); } // send multipart form data necesssary after file data... dos.writeBytes(lineEnd); dos.writeBytes(twoHyphens + boundary + twoHyphens + lineEnd); // Responses from the server (code and message) serverResponseCode = conn.getResponseCode(); String serverResponseMessage = conn.getResponseMessage(); Log.i( "uploadFile" , "HTTP Response is : " + serverResponseMessage + ": " + serverResponseCode); if (serverResponseCode == 200 ){ runOnUiThread( new Runnable() { public void run() { String msg = "File Upload Completed.\n\n See uploaded file here : \n\n" +uploadFileName; messageText.setText(msg); Toast.makeText(UploadToServer. this , "File Upload Complete." , Toast.LENGTH_SHORT).show(); } }); } //close the streams // fileInputStream.close(); dos.flush(); dos.close(); } catch (MalformedURLException ex) { dialog.dismiss(); ex.printStackTrace(); runOnUiThread( new Runnable() { public void run() { messageText.setText( "MalformedURLException Exception : check script url." ); Toast.makeText(UploadToServer. this , "MalformedURLException" , Toast.LENGTH_SHORT).show(); } }); Log.e( "Upload file to server" , "error: " + ex.getMessage(), ex); } catch (Exception e) { dialog.dismiss(); e.printStackTrace(); runOnUiThread( new Runnable() { public void run() { messageText.setText( "Got Exception : see logcat " ); Toast.makeText(UploadToServer. this , "Got Exception : see logcat " , Toast.LENGTH_SHORT).show(); } }); Log.e( "Upload file to server Exception" , "Exception : " + e.getMessage(), e); } dialog.dismiss(); return serverResponseCode; } // End else block } } |
Php script at server :
File : UploadToServer.php
<?php $file_path = "uploads/" ; $file_path = $file_path . basename ( $_FILES [ 'uploaded_file' ][ 'name' ]); if (move_uploaded_file( $_FILES [ 'uploaded_file' ][ 'tmp_name' ], $file_path )) { echo "success" ; } else { echo "fail" ; } ?> |
Show uploaded image at server :
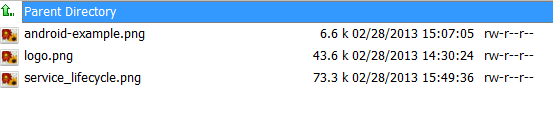
No comments :
Post a Comment